I wrote an higher order component in React to make the opening hours of a local shop more fancy. The UI emphasizes the current day with another background color. To make this happen the component needs to know which day we have so I wrap it with my hoc withToday().
The withToday() component needs 18 lines of code and makes use of the built-in Date object. All browsers should support it but nevertheless I decided to implement a fallback. I case the API does not work it returns null. In all other cases it returns an integer due to the current day.
import { useEffect, useState } from 'react'
export default function withToday(Element) {
return (props) => {
const [today, setToday] = useState(null)
useEffect(() => {
setToday(new Date().getDay())
}, [])
if (today === null) {
return null
}
return <Element {...props} today={today} />
}
}
Be aware that it returns 0 for Sunday. This is might be a pitfall because for example in Germany Monday seems to be the first day of the week.
Another important aspect is to create the instance in useEffect() otherwhise it will be some kind of cached. I tried without it and I realized that the day does not change. The empty dependency array as the second argument tells the hook to just render once when it gets mounted.
A really intersting mistake I made was to put the integer variable today in an conditional if statement.
if (!today) {
return null
}
The problem is the Sunday. Here my hoc returns o which is a valid integer. But in Javascript !0 returns true. For me this was a really good mistake to learn and improve my coding skills.
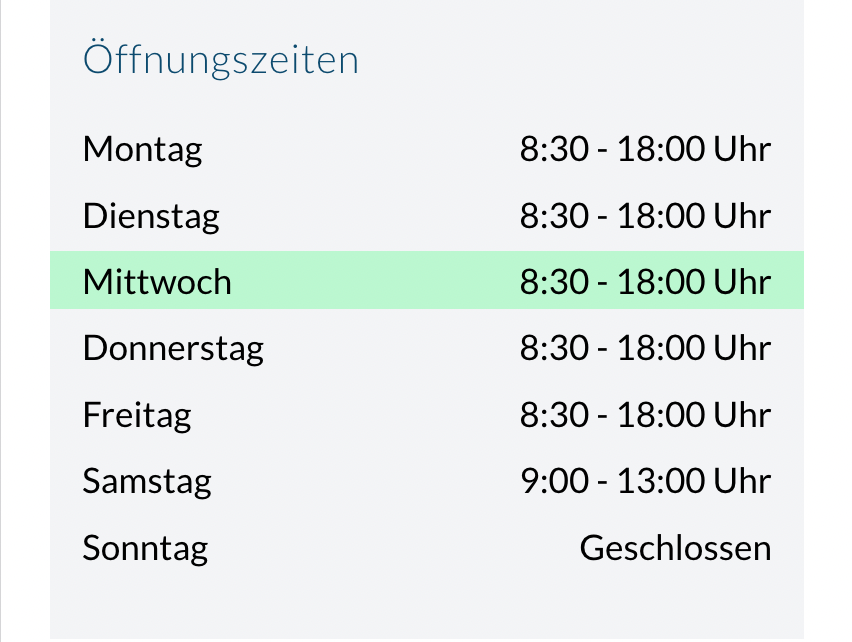
The same with the hoc. I don’t think that it was really that necessary to outsorce the logic here because I won’t need today in another component than the opening hours. Shure there is as well the Single-Responsibility-Pattern that tells you to keep components as little as possible. But I might dive deeper into this in another blog post.