I wrote a higher-order component in React to make the opening hours of a local shop more fancy. The UI emphasizes the current day with a different background color. To make this happen, the component needs to know which day it is, so I wrapped it with my HOC withToday().
The withToday() component needs 18 lines of code and makes use of the built-in Date object. All browsers should support it, but nevertheless, I decided to implement a fallback. In case the API does not work, it returns null. In all other cases, it returns an integer representing the current day.
import { useEffect, useState } from 'react'
export default function withToday(Element) {
return (props) => {
const [today, setToday] = useState(null)
useEffect(() => {
setToday(new Date().getDay())
}, [])
if (today === null) {
return null
}
return
}
}
Be aware that it returns 0 for Sunday. This might be a pitfall because, for example, in Germany, Monday is considered the first day of the week.
Another important aspect is to create the instance in useEffect(); otherwise, it will be cached. I tried without it and realized that the day does not change. The empty dependency array as the second argument tells the hook to run only once when it gets mounted.
A really interesting mistake I made was to put the integer variable today in a conditional if statement.
if (!today) {
return null
}
The problem is Sunday. Here, my HOC returns 0, which is a valid integer. But in JavaScript, !0 returns true. For me, this was a really good mistake to learn and improve my coding skills.
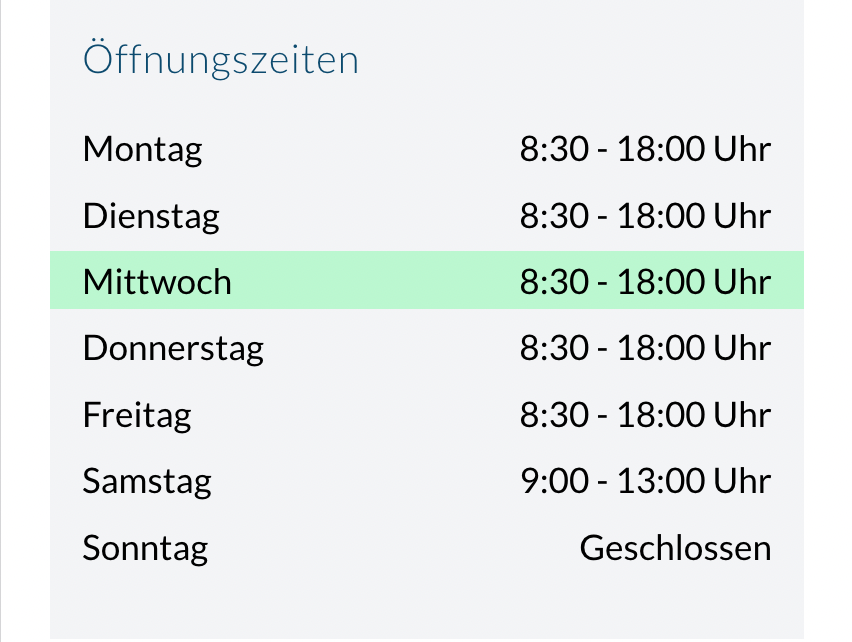
The same with the HOC. I don’t think it was really necessary to outsource the logic here because I won’t need today in any other component than the opening hours. Sure, there is also the Single-Responsibility Principle that suggests keeping components as small as possible. But I might dive deeper into this in another blog post.